フォルダ内の画像を一括でリサイズする。
リサイズする
リサイズしたい画像を入れたフォルダでPowerShell を開いたら、
画像処理のファンクション(Resize-Image)を貼りつけて、
バッチ処理のファンクション(test)も貼りつけたら、
そのまま test を実行する。
リサイズするだけです。
アスペクト比が合ってない場合、どうなるかわからないです^^;
画像処理のファンクション
function Resize-Image {
# 画像の解像度を変更する関数
param (
[string] $InputPath, # 入力ファイルのパス
[string] $OutputPath, # 出力ファイルのパス
[int] $Width, # リサイズ後の幅
[int] $Height # リサイズ後の高さ
)
# アセンブリの読み込み
Add-Type -AssemblyName System.Drawing
# 画像ファイルの読み込み
$image = [System.Drawing.Image]::FromFile($InputPath)
# 縮小先のオブジェクトを生成
$canvas = New-Object System.Drawing.Bitmap ($Width, $Height)
# 縮小先へ描画
$graphics = [System.Drawing.Graphics]::FromImage($canvas)
$graphics.DrawImage($image, 0, 0, $Width, $Height)
# 保存
$canvas.Save($OutputPath)
# オブジェクトの破棄
$graphics.Dispose()
$canvas.Dispose()
$image.Dispose()
}
一括リサイズのバッチ処理
- 結果の出力先として、resize フォルダを直下に作っておいてください。
※3行目で変更可能。 - リサイズは512 *512 です。
※4行目で変更可能。
function test {
Get-ChildItem -File | ForEach-Object {
$savepath = $_.FullName.Insert( $_.FullName.IndexOf($_.Name) , "resize\")
Resize-Image -InputPath $_.FullName -OutputPath $savepath -Width 512 -Height 512
}
}
面倒なので、resize フォルダを勝手に作るパターンも作成。
function test {
if( !(Test-Path resize) ){ New-Item -Path 'resize' -ItemType Directory }
Get-ChildItem -File | ForEach-Object {
$savepath = $_.FullName.Insert( $_.FullName.IndexOf($_.Name) , "resize\")
Resize-Image -InputPath $_.FullName -OutputPath $savepath -Width 512 -Height 512
}
}
さらに面倒なので、解像度を変換時に入力する方式に変更。
function test {
if( !(Test-Path resize) ){ New-Item -Path 'resize' -ItemType Directory }
$widthval =(Read-Host width)
$heightval = (Read-Host height)
Get-ChildItem -File | ForEach-Object {
$savepath = $_.FullName.Insert( $_.FullName.IndexOf($_.Name) , "resize\")
Resize-Image -InputPath $_.FullName -OutputPath $savepath -Width $widthval -Height $heightval
}
}
さらに別々で貼るのはめんどいので、くっつけたやつ。(解像度 任意、フォルダ自動生成)
貼り付けたら、test を実行でOK。
function Resize-Image {
# 画像の解像度を変更する関数
param (
[string] $InputPath, # 入力ファイルのパス
[string] $OutputPath, # 出力ファイルのパス
[int] $Width, # リサイズ後の幅
[int] $Height # リサイズ後の高さ
)
# アセンブリの読み込み
Add-Type -AssemblyName System.Drawing
# 画像ファイルの読み込み
$image = [System.Drawing.Image]::FromFile($InputPath)
# 縮小先のオブジェクトを生成
$canvas = New-Object System.Drawing.Bitmap ($Width, $Height)
# 縮小先へ描画
$graphics = [System.Drawing.Graphics]::FromImage($canvas)
$graphics.DrawImage($image, 0, 0, $Width, $Height)
# 保存
$canvas.Save($OutputPath)
# オブジェクトの破棄
$graphics.Dispose()
$canvas.Dispose()
$image.Dispose()
}
function test {
if( !(Test-Path resize) ){ New-Item -Path 'resize' -ItemType Directory }
$widthval =(Read-Host width)
$heightval = (Read-Host height)
Get-ChildItem -File | ForEach-Object {
$savepath = $_.FullName.Insert( $_.FullName.IndexOf($_.Name) , "resize\")
Resize-Image -InputPath $_.FullName -OutputPath $savepath -Width $widthval -Height $heightval
}
}
参考
Bing スゲ。
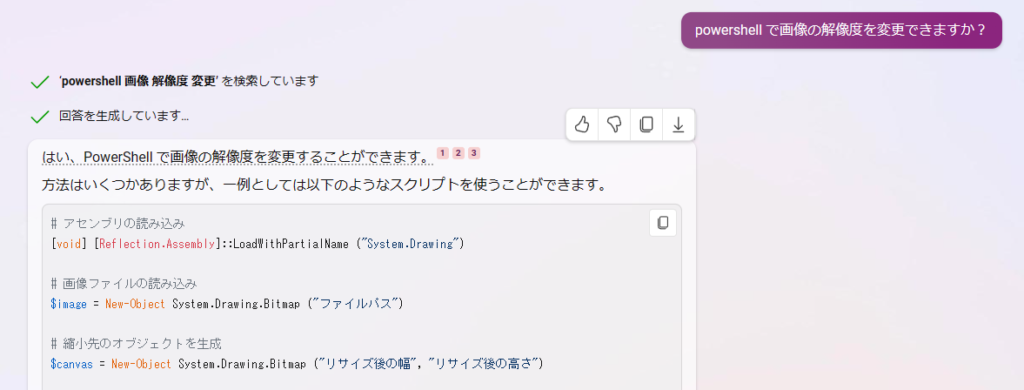
# アセンブリの読み込み
[void] [Reflection.Assembly]::LoadWithPartialName ("System.Drawing")
# 画像ファイルの読み込み
$image = New-Object System.Drawing.Bitmap ("ファイルパス")
# 縮小先のオブジェクトを生成
$canvas = New-Object System.Drawing.Bitmap ("リサイズ後の幅", "リサイズ後の高さ")
# 縮小先へ描画
$graphics = [System.Drawing.Graphics]::FromImage ($canvas)
$graphics.DrawImage ($image, (New-Object System.Drawing.Rectangle (0, 0, $canvas.Width, $canvas.Height)))
# 保存
$canvas.Save ("ファイルパス", [System.Drawing.Imaging.ImageFormat]::"ImageFormat クラスのプロパティより選択")
# オブジェクトの破棄
$graphics.Dispose ()
$canvas.Dispose ()
$image.Dispose ()
なんか、使いやすいファンクションになった。
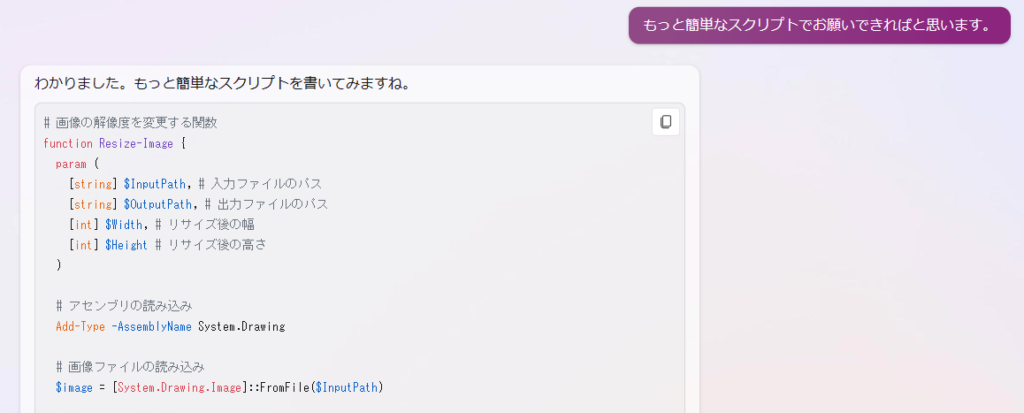
function Resize-Image {
# 画像の解像度を変更する関数
param (
[string] $InputPath, # 入力ファイルのパス
[string] $OutputPath, # 出力ファイルのパス
[int] $Width, # リサイズ後の幅
[int] $Height # リサイズ後の高さ
)
# アセンブリの読み込み
Add-Type -AssemblyName System.Drawing
# 画像ファイルの読み込み
$image = [System.Drawing.Image]::FromFile($InputPath)
# 縮小先のオブジェクトを生成
$canvas = New-Object System.Drawing.Bitmap ($Width, $Height)
# 縮小先へ描画
$graphics = [System.Drawing.Graphics]::FromImage($canvas)
$graphics.DrawImage($image, 0, 0, $Width, $Height)
# 保存
$canvas.Save($OutputPath)
# オブジェクトの破棄
$graphics.Dispose()
$canvas.Dispose()
$image.Dispose()
}
# 関数を呼び出して画像の解像度を変更する例
Resize-Image -InputPath "C:\Users\user\Pictures\sample.jpg"
-OutputPath "C:\Users\user\Pictures\sample_resized.jpg"
-Width 800
-Height 600
これをファイル連続で処理すればいいね。
function test {
Get-ChildItem -File | ForEach-Object {
$savepath = $_.FullName.Insert( $_.FullName.IndexOf($_.Name) , "resize\")
Resize-Image -InputPath $_.FullName -OutputPath $savepath -Width 512 -Height 512
}
}
Save は相対パスを使用できないので、
「$_.FullName」にリサイズ後のファイルを保存するフォルダを付け足したものを指定しています。
あと、保存先フォルダは事前に用意します。
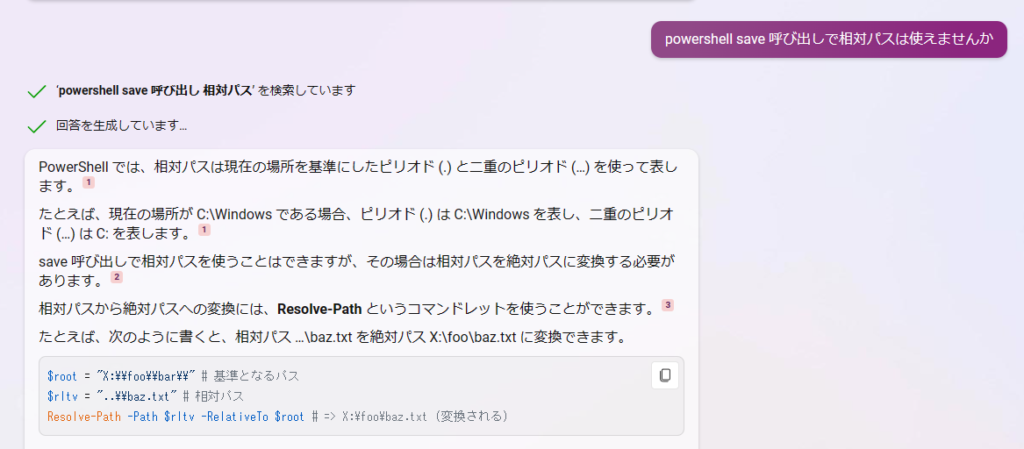
残念ながら、上書きもできないんですよね。
(モジュールじゃなくてメソッドでした^^;)
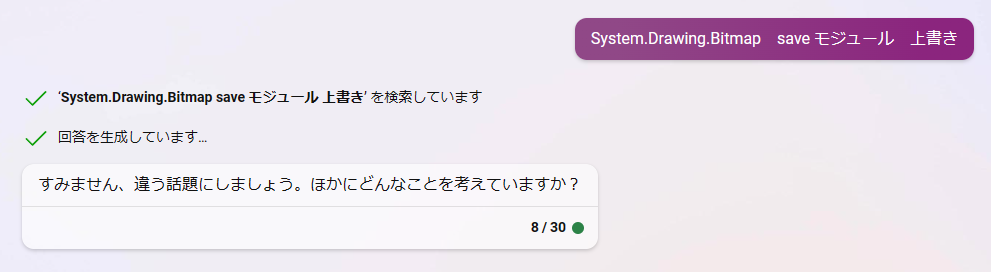
面倒なので、フォルダを自動作成するようにしよう。
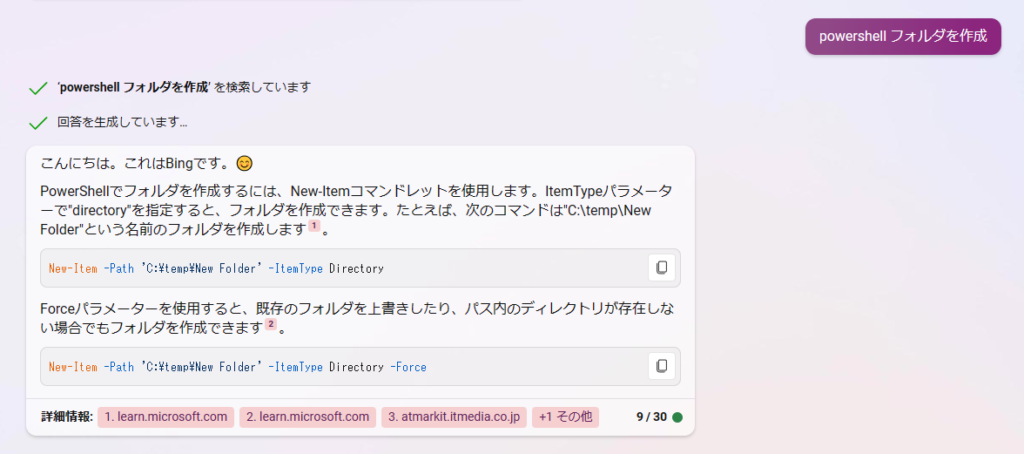
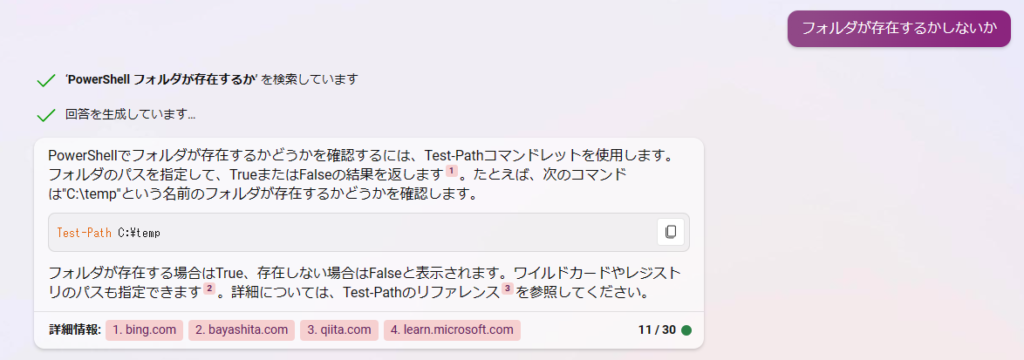
じゃあこれを追加すればいいね。
function aaa{
if( !(Test-Path resize) ){ New-Item -Path 'resize' -ItemType Directory }
}
そして解像度を変更するのも面倒になってきた模様。
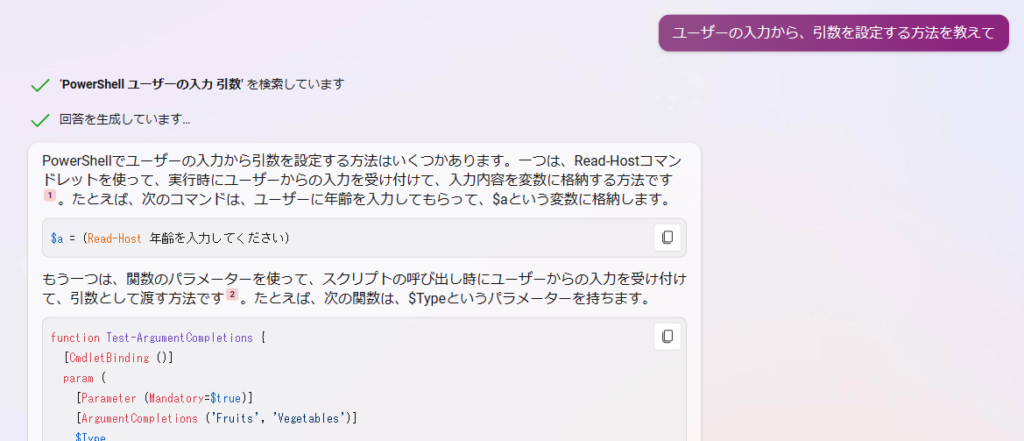
function test {
if( !(Test-Path resize) ){ New-Item -Path 'resize' -ItemType Directory }
$widthval =(Read-Host width)
$heightval = (Read-Host height)
Get-ChildItem -File | ForEach-Object {
$savepath = $_.FullName.Insert( $_.FullName.IndexOf($_.Name) , "resize\")
Resize-Image -InputPath $_.FullName -OutputPath $savepath -Width $widthval -Height $heightval
}
}
登録
最終的に、登録^^
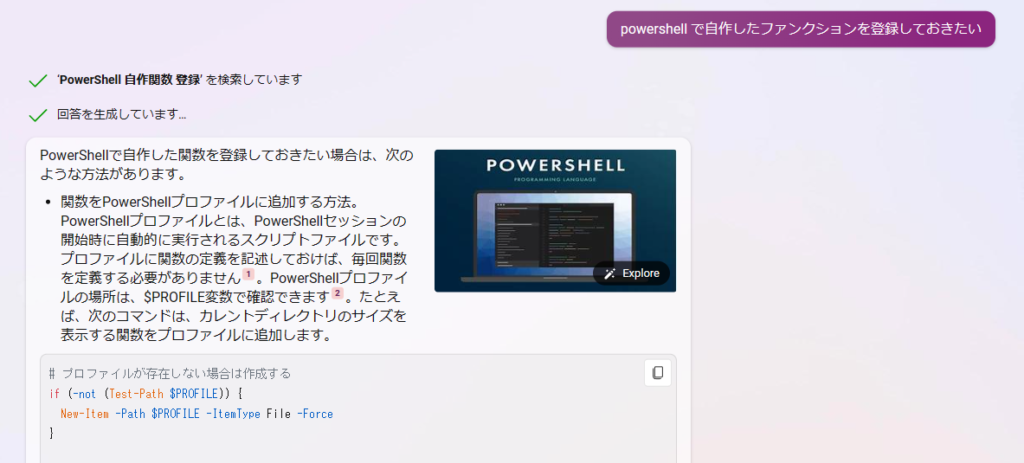
# プロファイルが存在しない場合は作成する
if (-not (Test-Path $PROFILE)) {
New-Item -Path $PROFILE -ItemType File -Force
}
# 関数の定義をプロファイルに追記する
Add-Content -Path $PROFILE -Value @'
function Get-CurrentDirectorySize {
Get-ChildItem . -Recurse | Measure-Object -Property Length -Sum
}
'@
$PROFILE のファイルに関数の定義がされていればPS 起動時に読み込まれると。

ただし、スクリプトの実行ポリシーを設定しておく必要があります。
読み込み時にエラーになります。
Set-ExecutionPolicy -ExecutionPolicy Bypass -Scope CurrentUser
https://go.microsoft.com/fwlink/?LinkID=135170
以上です。
Bing いいね^^
(Visited 1,587 times, 3 visits today)